Data types in R
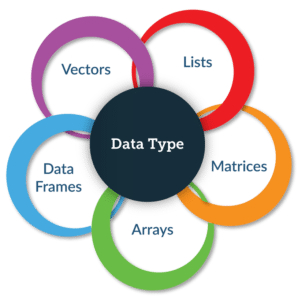
Data types in R-SoftwareThe elements of a data can be a numeric value, a character or a logical value.The types of data are-
- Vectors
- Lists
- Matrices
- Arrays
- Factors
- Data frames
(1) Vectors – It is a sequence of multiple data of same types, that is, numeric, character or logical. Few examples are shown below-
Code
Age <-c(22,21,32,61,5) ## it is vector of numeric type.
class(Age)
Names<-c(“manvir”,”yash”,”alestaa”,”rohit”,”raj”)## it is a vector of character type consisting the name of five individuals
class(Names)
Output:
Age 22 21 32 61 5
“numeric”
Names “manvir” “yash” “alestaa” “rohit” “raj”
## this code class(variable) will tell you the class of the elements in the vector, that is, they are character or numerical or logical.
“character”
(2) Lists- list is a sequence of different types of elements like numerics, characters and logical values and etc. Unlike a vector, different elements can be stored within a list without making them loose their originality.
Code-
L<-list(“a”,”b”,2, TRUE)
## we have stored 3 different types of elements- numeric, character and logical together.
Output-
[[1]] [1] “a” [[2]] [1] “b” [[3]] [1] 2 [[4]] [1] TRUECode-str(L) ## this code will tell us the type of each element in the list.
Output-List of 4
$ : chr “a”
$ : chr “b”
$ : num 2
$ : logi TRUE
## “chr” is character, “num” is numeric and “logi” is logical.
So we can see that we can store different type elements together in a list and elements do not lose their originality which is not possible in a vector.
(3) Matrices – A matrix is a combination of multiple vectors with same types of elements. It is an object in which elements are arranged in a 2-D rectangular layout. In R, a matrix is created using the function matrix().
Arguments- matrix(data, nrow, ncol, byrow = FALSE, dimnames = NULL)
##Data will be the vector of same type elements of whose matrix we want to create.
## nrow and ncol will be tell R the number of row and column of the matrix.
## by default , in R the data will be arranged in matrix format column wise. If you want to arrange the data in the matrix row wise, set byrow= TRUE.
## suppose the matrix has two rows and two columns. The function dimnames is used to name the rows and columns of the matrix. By default, it is null and the matrix’s dimension has no names
Suppose you want to create a 1*3 matrix, that is, a matrix with 1 row and three columns. The rows will show the amount you owe to three individuals – a, b, c. The amount is 5, 10 and 15 respectively.
Code:
matrix(c(5,10,15), nrow=1, ncol=3, byrow= TRUE)
## we want the data to be row wise so byrow= TRUE (note that due to just one row,the argument byrow=T does not makes sense)
Output
[,1] [,2] [,3] [1,] 5 10 15
## we can clearly see that since we did not specify the dimnames so they are nameless.
## now specifying the dimnames as well. It is of list data type.
Code
row<-c(“amount”) ## to specify the row name of the matrix
col<-c(“a”,”b”,”c”) ## to specify the column names of the matrixmatrix(c(5,10,15), nrow=1, ncol=3, byrow=TRUE,dimnames = list(row,col))
Output
a b c
amount 5 10 15
## the same matrix but with dimension names specified.
Similarly you can create matrix of other dimensions of your choice and name them accordingly.
(4) Arrays- Arrays are similar to matrices but it can have more than two dimensions. To create an array, we call the array function and input a vector of values and a vector of dimensions. Unlike a matrix, it can have more than two dimensions. We also have the option of naming the dimensions.
Arguments – array(data = NA, dim = length(data), dimnames = NULL)
The data in an array will always be shown in column wise. So we will have to put the data accordingly.
## data- we will input the vector of the data whose array we want to create.
## dim- this argument will help us to input the dimensions of the arrays. It can have more than two dimensions.
## dimnames- this argument will help us to name the dimnesions of the array.
Suppose you want to create an array of marks in Mathematics and Physics of three students – a, b and c in class 10 and 12 so as to see their progress in each subject.
Code-
students<-c(“a”,”b”,”c”)
subjects<-c(“mathematics”,”physics”)
standard<-c(“class 10”, “class 12”)
## these vectors are created just to name the dimensions of the array
array(c(52,45,36,28,45,46,78,79,45,46,12,36),dim=c(3,2,2), dimnames=list(students,subjects,standard))
## this code will create an array of dimension 3(rows)*2(columns)*2(number of matrices) displaying the marks of the students in maths and physics in class 10 and 12 . It will create 2 matrices of 3 rows and 2 columns.
Output-
, , class 10
mathematics physics
a 52 28
b 45 45
c 36 46
, , class 12
mathematics physics
a 78 46
b 79 12
c 45 36
## Note that the data in the matrix gets fitted column wise by default so put the data accordingly.
(5) Factors – factors are the data objects which are used to categorize the data and store it as levels. They can store both integers and strings. Factors are created using the function factor() after taking a vector as an input.
Code-
data<-c(“male”,”female”,”male”,”male”,”female”,”male”)##input vector
factor(data)## this code will tell us the categories/ levels of the vector data.
Output-male female male male female male
Levels: female male
## the vector data contains six characters of two types – male and female.
(6) Data frame- A data frame is a table like structure in which each column contains values of one variable with “n” number of rows. The number of rows in each column should be equal otherwise this function will not work. Data frame is created using the function data.frame().
Suppose you want to create a table like structure for 5 people- a, b, c, d and e specifying their age and gender. You have all the required information with yourself.
Code-
gender<-c(“female”,”male”,”male”,”female”,”male”)
name<-c(“a”,”b”,”c”,”d”,”e”)
age<-c(18,15,22,14,16)
data.frame(name,age,gender)
Output-
name age gender
1 a 18 female
2 b 15 male
3 c 22 male
4 d 14 female
5 e 16 male
## you can use more variables as well and ad more columns and row to the table. Note that the number of elements in each column should be same otherwise this function will not work.
We hope this article will help you. Try creating some arrays, matrices of different dimensions too by yourself. Also practice the other data types. Happy learning.
I definitely wanted to send a brief note to be able to say thanks to you for some of the awesome strategies you are giving out on this site. My long internet lookup has now been rewarded with useful ideas to write about with my friends and classmates. I would express that we website visitors actually are very fortunate to exist in a useful website with many perfect individuals with beneficial basics. I feel rather lucky to have come across your entire web pages and look forward to some more excellent times reading here. Thanks a lot again for everything.
Thanks a lot for giving everyone an extraordinarily splendid opportunity to read in detail from this site. It is always so sweet and as well , jam-packed with a great time for me personally and my office fellow workers to visit the blog minimum 3 times weekly to read through the newest secrets you will have. And lastly, I’m just always motivated with all the outstanding tips served by you. Selected two tips in this posting are essentially the very best I have ever had.
Thanks for your entire hard work on this web page. Kate enjoys doing research and it is easy to see why. A lot of people hear all about the lively form you produce very useful suggestions through the web site and therefore encourage contribution from visitors on the concept so our child has always been being taught a whole lot. Have fun with the rest of the year. You’re conducting a powerful job.